Enabling Developers To Ship Cloud-Native Applications Faster
For cloud-native applications, moving code from a developerâs local machine to a staging or testing environment is rarely straightforward. In a common setup, developers first commit their code to a version control system. Then, a CI system builds a container image with the latest changes. Finally, a CD system (like Argo CD ) deploys that image to a Kubernetes cluster. It is only after all of this that developers are able to test out their changes. This process, repeated multiple times per feature, can be really slow.
Of course, this isnât the only workflow. Some teams use remote development environments where code is compiled directly in the cluster with file synchronization. Others spin up ephemeral environments in CI pipelines for automated end-to-end testing. From this variety of dev loops, itâs clear thereâs no one-size-fits-all but in nearly every case, the friction from code-to-test remains a shared pain.
We built mirrord to change that. mirrord shifts testing left by letting developers run their code in a real Kubernetes environment instantly, without needing to rebuild or redeploy. It allows developers to use a shared cluster for development, removing the need for isolated, costly personal environments. Instead of waiting for CI pipelines or clashing in shared dev or integration clusters, each developer can connect to the same Kubernetes environment and test their changes safely and independently. This avoids the usual environment collisions while reducing the iteration time from 15â30 minutes per change down to just a few seconds.
In this blog Iâll walk you through how this works!
Problems With Shifting Application Testing Left
Shifting left in software development means moving processes (like testing) earlier in the development lifecycle. This approach helps catch issues early rather than later in the cycle. The earlier you identify issues, the easier they are to fix, and the faster you can ship. This is why more and more organizations today are recognizing the importance of enabling developers to test their code as early in the development cycle as possible. However, this is currently challenging for several reasons:
- Developers often lack the necessary Kubernetes expertise to deploy and test their code on a local cluster.
- Shared environments can cause delays. When staging is used for pre-release validation, it may be unavailable for testing other features. Thereâs also the risk of a developerâs code breaking something in the shared environment, leading to further delays.
- Setting up a separate environment for each developer to avoid these issues can be costly and create significant maintenance overhead.
Shortening the Development Loop With mirrord
We built mirrord to make developing modern applications significantly faster. It does so by allowing developers to test their code in a real Kubernetes environment without the need for repeated deployments, thus significantly shortening the development loop. Hereâs how it works:
mirrord consists of two main components:
- mirrord-layer: This is the local component that runs alongside your application process. It intercepts system calls made by the process locally and redirects them to the remote Kubernetes cluster.
- mirrord-agent: This is the remote component that runs as a pod in your Kubernetes cluster. It handles the actual interaction with the clusterâs resources and communicates with the mirrord-layer.
When you start your application with mirrord, your local process is seamlessly connected to the Kubernetes environment. mirrord acts as a proxy between your machine and the cluster, intercepting and redirecting your applicationâs inputs and outputs so that it behaves as if it’s running inside the cluster, even though it’s still running locally. This gives your code access to real traffic, services, files, and configurations from the cluster without requiring any deployment.
Hereâs how it works in detail:
- Incoming traffic to the target pod in the cluster is mirrored to your local process. This means you can test your changes with real traffic without affecting the actual pod or the staging environment.
- Outgoing traffic from your local process is routed through the mirrord-agent, making it appear as if itâs originating from the cluster. This also allows your local process to interact seamlessly with other services in the cluster without you having to run them locally.
- mirrord also allows your local process to steal some (or all) of the traffic coming to service youâre developing from the cluster. This means that you can have your locally written code answer requests coming to the staging endpoint and see how it behaves. Weâll soon see this in action in the next section.
- mirrord also redirects file reads and writes to the remote podâs file system, ensuring that your local process interacts with the same files as the deployed application.
- It also merges the environment variables of the remote pod with those of your local process, ensuring that your application has access to the same configuration and secrets as it would in the cluster.
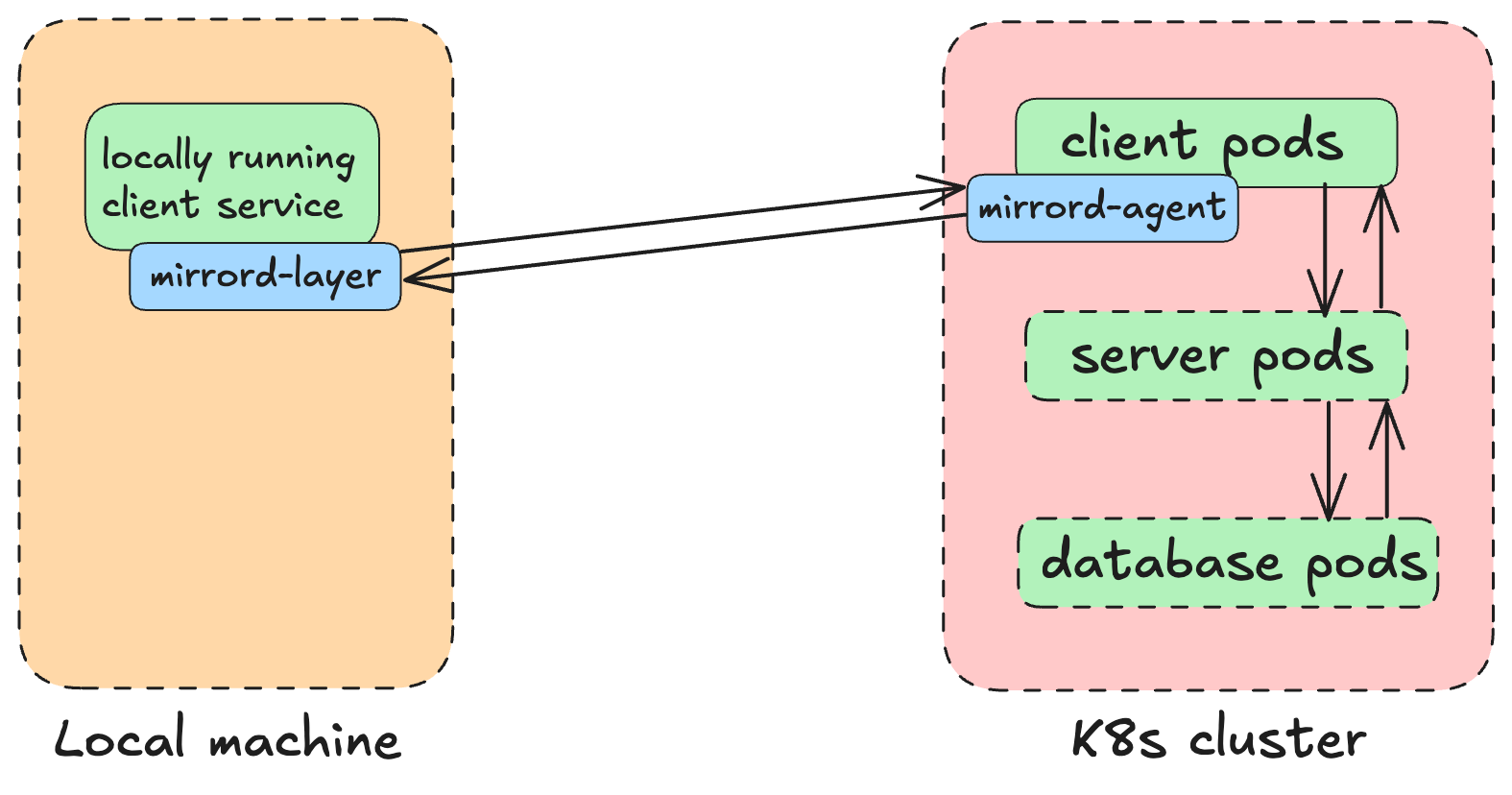
All of this means that developers can test their code in a real world environment without the overhead of repeated deployments. Letâs walk through a step-by-step tutorial to see this in action!
Preparing our Kubernetes cluster
The first thing you need to enable your developers to start using mirrord for development is to have a Kubernetes cluster ready. This cluster should already have the application your developers will be working on deployed. If youâre using a shared development, testing, or staging cluster, you’re likely already set up for this. In addition to the application itself, the cluster also needs to have the mirrord operator installed.
For this blog, weâll use a classic todo list application as our example. It includes a React frontend, a Node.js backend, and a PostgreSQL database. You can find the code, along with the Kubernetes manifests needed to deploy it, in this GitHub repository .
Make sure to deploy an ingress controller on the cluster before deploying the app. I used the nginx-ingress-controller , but you can use any alternative that you prefer.
Installing the mirrord Operator
To install the mirrord operator, youâll first need a license key. You can generate one from your MetalBear account . Once you have your key, follow these steps to install the operator on your cluster:
Donât forget to update the
license.key
field invalues.yaml
with your actual license key before installation.
$ helm repo add metalbear https://metalbear-co.github.io/charts
$ curl https://raw.githubusercontent.com/metalbear-co/charts/main/mirrord-operator/values.yaml --output values.yaml
$ helm install -f values.yaml mirrord-operator metalbear/mirrord-operator
The mirrord operator is what enables multiple developers in your organization to connect to the same cluster at the same time and use it for development while avoiding any clashes. Each developer gets an isolated path to develop and test their changes. This eliminates the need to duplicate infrastructure or spin up costly, time-consuming ephemeral environments.
Developing a Microservice With mirrord
Once your cluster has both the application and the mirrord operator installed, your developers are ready to start developing against it. Hereâs what our deployed todo list application looks like:
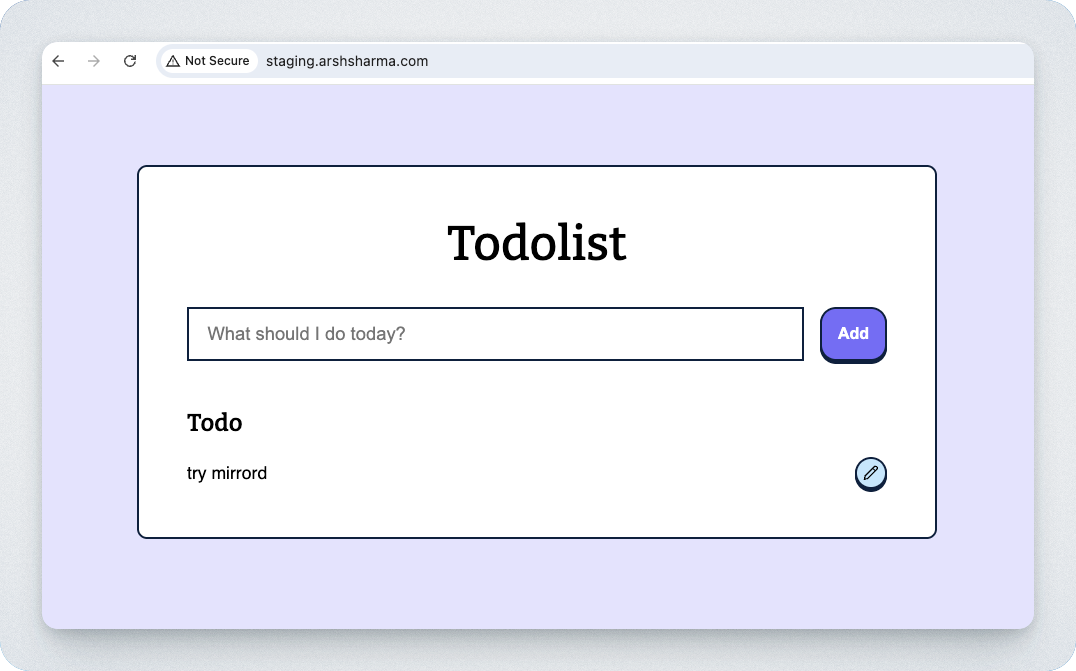
Right now, if youâll see, thereâs no way for users to delete their added todos. Imagine youâre a frontend developer tasked with adding a âdeleteâ button next to each todo item. Youâve written the code for this feature and are ready to test it.
But hereâs the catch: the frontend depends on a backend API, the database, and other shared environment configuration. All of this isnât available locally. So traditionally, youâd have to commit your code, wait for the CI process to complete, and then deploy it to the staging environment. Youâd then be able to test the feature, possibly run some end-to-end (e2e) tests, and if you find bugs, youâd repeat the entire process. This cycle can be time-consuming and frustrating. We built mirrord so you can avoid all of that!
Installing the mirrord CLI
The first thing you need to do is install the mirrord CLI on your local machine. mirrord also offers extensions for various code editors, but for this tutorial, weâll focus on the CLI.
You can install the CLI on macOS by running:
brew install metalbear-co/mirrord/mirrord
For other platforms, detailed instructions can be found in our documentation . Weâve made the setup experience with mirrord as frictionless as possible. You should be able to get started in under 5 minutes.
Once installed, youâll need the name of the target deployment in the Kubernetes cluster running the frontend service.
The GitHub repo for the todo list app has Helm charts along with instructions on how to deploy the application to a cluster!
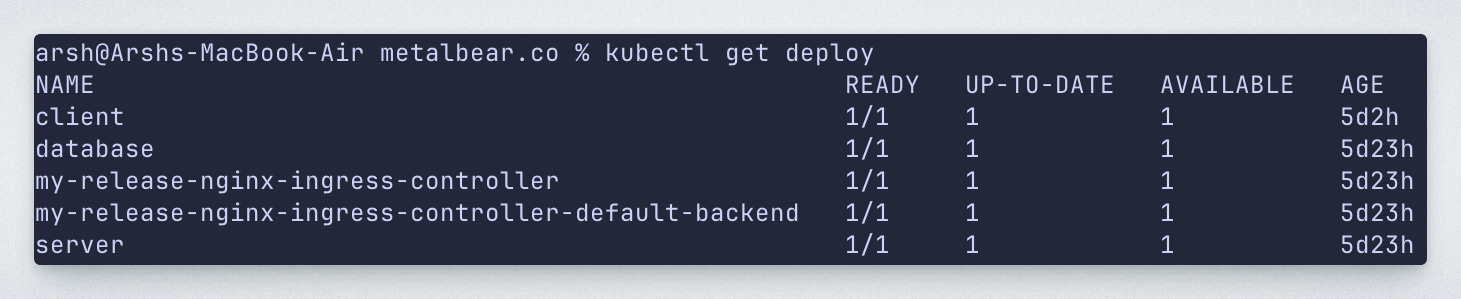
For us, thatâs client
.
Running Your Application With mirrord
Once we know which pod is running the service we want to connect to, we can simply run the following command to launch the mirrord-agent
pod on our staging cluster and start testing our changes:
Before you do this make sure to navigate to the
client
folder locally and runnpm install
to install dependencies.
(todolist/client) $ mirrord exec --target deployment/client npm start
Running the above command starts the client (frontend) service locally while mirroring traffic from the target deployment in the Kubernetes cluster. This allows you to test your changes in a real environment without actually deploying them.
When you interact with this service (currently running on localhost
), you might notice that adding a todo item or the delete functionality doesnât work. Thatâs because the application is designed to be accessed via an ingress URL, specifically, the staging FQDN
configured in the ingress resource. On top of that the code for the delete functionality is also currently commented out.
To test the app as intended, weâll access it through its staging URL, where both services are deployed behind the ingress. However, by default, mirrord only mirrors traffic to your local machine while the original pod continues serving requests. If we want to test our newly written changes on the staging URL, we need our locally running code to handle incoming requests instead of the pod. This can be done by enabling steal mode .
Enabling Steal Mode
As a frontend developer, you shouldnât have to run the backend or database services locally to test your changes. Instead, you can leverage the ones running in the staging environment.
To do this, enable the steal
mode in mirrord. This mode allows your local code to serve requests reaching the pod running in the staging cluster.
To enable steal mode, create the following mirrord configuration file at the root of the repo:
# mirrordconfig.json
{
"target": "deployment/client",
"feature": {
"network": {
"incoming": "steal"
}
}
}
Now, run the following command to test your local code in the staging environment:
If you’re following this tutorial step by step, make sure to add the code responsible for the delete functionality. You’ll find it commented out in these lines here .
(todolist/client) $ mirrord exec -f=../mirrordconfig.json npm start
And voila! Now, when you visit the URL where the application has been deployed on the staging cluster (for me, thatâs staging.arshsharma.com
in the screenshot), youâll notice that the code thatâs actually serving those requests is the one on our local machine which has the delete functionality!
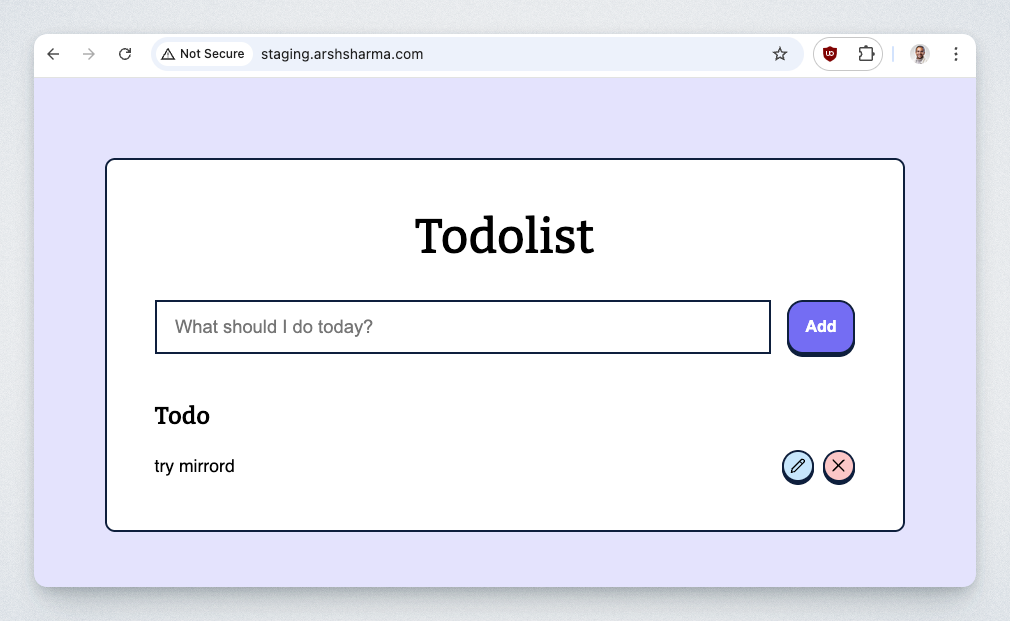
You can now test this feature, play around with it, or even run e2e tests against the staging URL like you would normally. All of this without having to commit or deploy your code!
Faster Development Loop With mirrord
I think you can now start to see how mirrord significantly speeds up the traditional development loop by reducing the time it takes for developers to test their code. Being able to connect your local code to a staging environment instantly allows developers to catch and fix bugs much faster. Hereâs what a developerâs workflow can look like with mirrord:
- Write code â Make changes to your application locally.
- Start mirrord â Run
mirrord exec
to connect your local process to the staging environment. - Test instantly â See your changes live, interacting with real services and traffic without redeploying.
- Debug and iterate â Identify and fix issues faster by testing in a real environment.
- Commit and deploy â Once everything works as expected, push your changes and move forward with the confidence that things wonât break :)
So if youâre ready to enable your team to ship faster try mirrord for free or reach out to us âweâd love to help you level up your dev workflow!